Around thirty days ago we had solar panels installed \o/. I think it's useful
for other people who are thinking about solar to have more information, so this
is a bit of a summary of how things have gone for us so far.
Ordering and installation
We ordered back at the end of November 2021 after getting some quotes from
local installers and E.ON. These are the rough quotes we got from E.ON (all the
quotes include the 5% VAT that was valid at the time):
- 15x390W panels (5.85kWp): £6400 (37%)
- 15x390W panels (5.85kWp) plus 5.2kWh battery: £9600 (59%)
- 11x390W panels (4.29kWp) plus 5.2kWh battery: £8600 (52%)
- 15x390W panels (5.85kWp) plus 8.2kWh battery: £10400 (63%)
kWp is "kilo Watt peak", the maximum output under certain conditions. The
percentages at the end are the claimed potential energy independence, so an
idea of how much of the electricity we'd potentially make use of vs export.
The local installer quotes were a bit more expensive, and crucially for us E.ON
were offering 3 years interest free credit which made it a lot more affordable
and meant we could go with the most expensive option, which otherwise would've
been tricky. Given that having a battery and more panels means the payback time
should be much smaller, that's a big advantage.
Systems over around 4kWp have to have permission from the Distribution Network
Operator (DNO) before they are installed. This application is undertaken by the
installers, but could take up to around 12 weeks to be approved - this was a big
part of the delay between our order and the installation. There was also a
delay of around three weeks because of supply problems getting the batteries
from China. That delay ultimately worked in our favour though, because the
installation happened after the change to 0% VAT. I dread to think what the
lead time is these days though.
The installation itself was pretty straightforward. The scaffolding was
erected, including having to block our back door, grr. The same day E.ON told
us they were running behind because installers had been off with covid... so we
had another week delay, grr. Once they were here though, the installers were
great. There were some chaps dealing with the roof fittings on the first day,
and an electrician starting the work with the inverter and battery as well. The
battery had a small dent in the top and the electrician said that he'd been
told not to use it, so we went along with that. He wasn't sure when we'd be
able to get a new one, given the delays they'd been having, but in the end
managed to get a new one the next day. On the second day the remainder of the
DC wiring run was completed, the panels themselves were installed and the full
system commissioned. Great.
The only bit that was annoying was when one of the roof installers showed me
the panels and pointed out how they'd made the effort to put the panels on the
roof so there was still space for another two in the future if we wanted. That
was a surprise because I'd thought we were filling the roof completely, based
on their measurements. The scaffolding came down the next day, it's unlikely
we'll be getting an extra two panels to fill the gap.
The system and data logging
The inverter and battery we have are a GivEnergy
Giv-HY 5.0 inverter and a Giv-Bat 8.2. These models are no longer available,
there are now gen 2 inverters and 9.2kWh batteries.
This is the inverter (top), battery (bottom), and generation meter (top right):
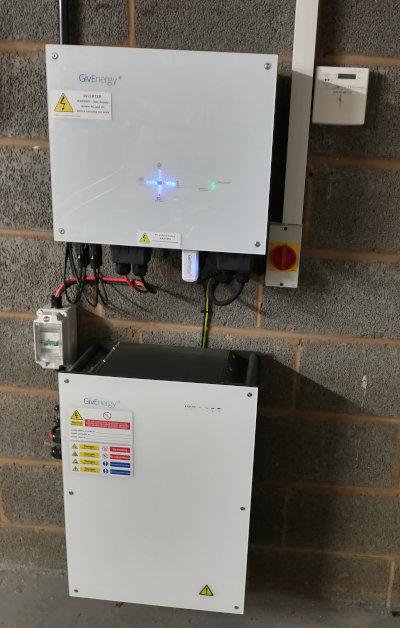
The inverter comes with a WiFi dongle that acts as an access point in the first
instance, so you can configure it to connect to your home WiFi, to send data up
to the cloud. We got access to two mobile apps that use the data - one from
E.ON and one from GivEnergy. The E.ON one gives a nice overview of different
generation/usage views, and also offers to integrate with other gadgets like
controllable lighting. The one from GivEnergy gives a similar view of energy,
but also offers some control features about how the battery should be used.
There are a few options, the obvious/easy difference is that in Summer you want
to charge the battery up in the day and use it at night, whereas in Winter you
may want to charge the battery overnight on a cheap rate, then use it in the
day when you aren't getting sufficient solar generation.
I have both apps installed, but typically use neither of them. Instead, I grab
my own data and save it to InfluxDB. Happily, the inverter has a modbus
interface which you can query to get lots of data. Even more happily, some
people have already put the effort in to get this working in a very easy way. I
looked at two solutions,
givenergy_modbus and
giv_tcp.
givenergy_modbus is a Python module that allows you to very easily query the
data from the inverter and battery and then do what you want with it. I like it
because I can write the code to do what I want - logging to InfluxDB.
giv_tcp is a docker image that contains components to query data from the
inverter and battery, and then... publish them to MQTT. Yep, this has Mosquitto
included in the image, which made me smile.
I believe there is also a "proper" HomeAssistant plugin nearing completion, but
I haven't looked at it because I don't currently use HomeAssistant.
The code I put together for using givenergy_modbus is on
github.
Family friendly monitoring
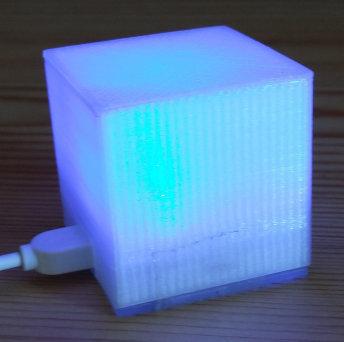
I have a lovely dashboard with all of the data that I'm interested in, but it's
not much use for others in the family. I've also got an MQTT enabled ambient
orb, and each time I take a measurement from the inverter the colour of the orb
is updated. The different states are:
- Green - battery is charging
- Red - battery is discharging
- Blue - battery is no charging (most likely exporting to grid)
- Pink - we are importing from the grid
In each case, the brightness of the colour indicates the intensity of the
state.
The orb sits in the hall where everyone can see it, and honestly I think it's
fairly useful during the day at least.
Other logging
I'm publishing spot generation values and AC frequency and voltage values to
solar/1d4a3c4f-493c-405a-b7c5-a6c558d2b6ab/#
on my test.mosquitto.org
MQTT
broker.
I've also recently set up an twitter bot
@ralight_solar to publish generation
graphs in a similar manner to @edent_solar.
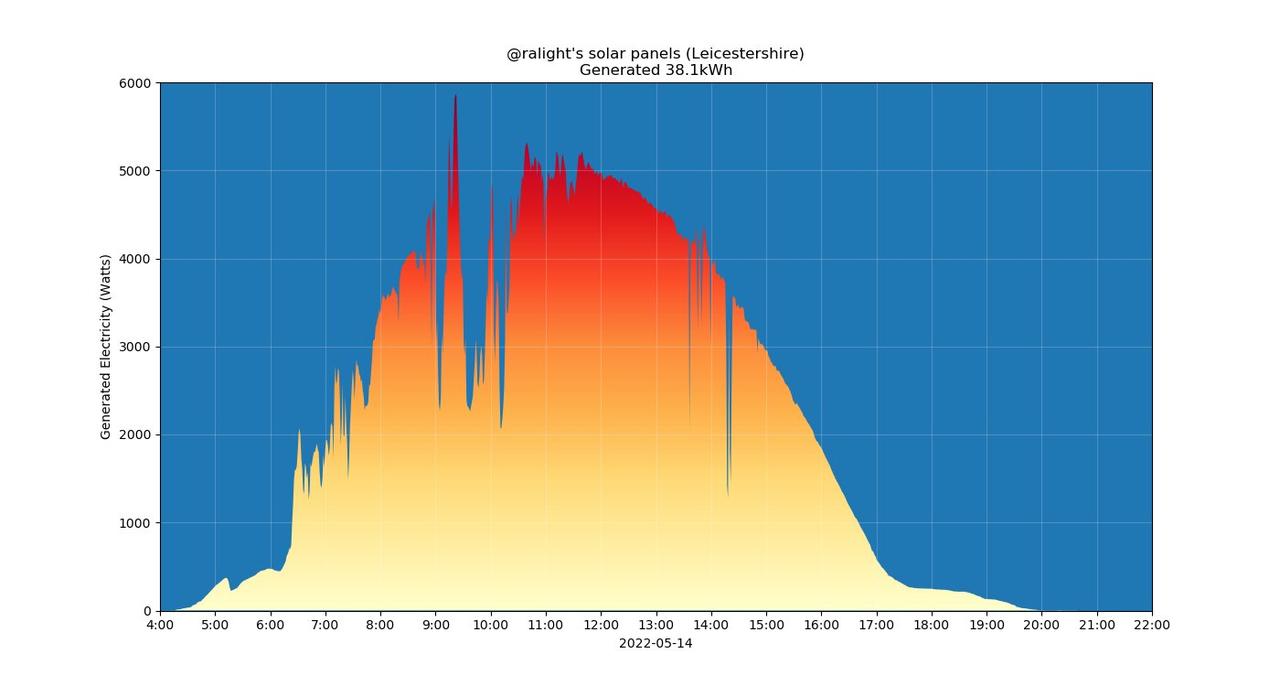
Generation, usage, and export
Over the last 30 days we've generated 760kWh in total, with the best generation
day producing 38.7kWh and the worst producing 7.8kWh.
This is what generation looked like over that time:
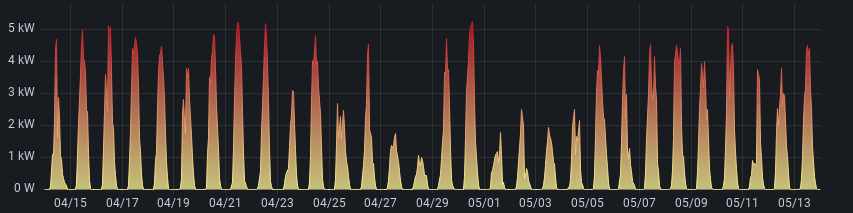
We've imported 8kWh during daytime, and 62kWh during nighttime.
Our hot water only comes from electricity and we are a large household, so we
have higher than typical usage. We used around 470kWh in the 30 days (and
barely any gas, by comparison). The numbers are not as accurate here, because
the inverter doesn't provide daily load demand figures unlike the daily
generation numbers.
It's difficult to make proper cost comparisons because we have an economy 7
meter and so pay different rates during the day and the night, but also because
we have changed when we use electricity. Using an average price of 25p/kWh for
the day and night, we would have been charged £117.50 without solar (ignoring
daily standing charges). The real cost is more like £17.50.
I'd still like to reduce the usage further. We've had a few days with
practically zero import and think that should happen more often. The biggest
cause of import is still down to hot water during the night. I'd like to get a
solar diverter like an iBoost to ensure we get as much generation pumped into
the hot water during the day, but I'm not sure whether the solutions that exist
right now are enough - I want to be able to guarantee that we have hot water in
the morning. If there was sufficient generation during the day then there's no
need to turn the hot water on at the night at all. I'm not sure the built in
thermostats are good enough for what I want.
We exported 364kWh. We don't yet have a smart meter so can't easily get an
export contract, but if we were with Octopus and on their 7.5p/kWh tariff then
that would have produced £27.30.
Total saving for the month, including the unrealised export value would be
around £127.30. I'm fairly happy with that.
Limitations
The biggest surprise for me was how the inverter works in different situations.
The inverter is rated at a nominal 5kW AC output which is obviously less than
our peak 5.8kW output. Our generation is clipped at 5.2kW. This isn't a
massive problem, because if we've got that much generation we've already
producing more than we'd be using - it does limit the export amount somewhat
though.
The exception to this situation is if the battery is still charging. In that
case, we can dump 2.5kW max into the battery and still pull up to the limit of
the panels. You can see that in this example, where the output is >6kW until
the battery charges at which point the output clips.
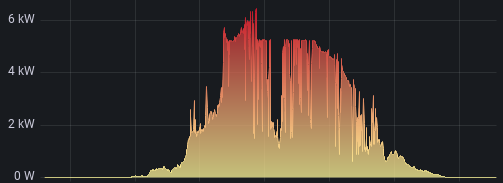
The other slight annoyance is that the inverter can only draw 2.5kW from the
battery at once. This is annoying because there are plenty of devices around
the home that draw >2.5kW - in particular for us, the hot water immersion. So
if we use the immersion at night, it costs money regardless of how much battery
capacity is available. Apart from the days where there were very low generation
and hence we needed lots of water heating at night time, a great deal of the
import we had was from the excess use over 2.5kW, even if the battery still had
sufficient capacity. I'm hoping a solar diverter can add a ~2.5kW limit for
night use when we get one. In the meantime, I only need ~700W generation to be
able to use the immersion during the day, assuming I have some battery charge.
The second gen of the inverter, available now, can draw 3.6kW from the battery,
so it's not a problem there.
Battery
We've had 4 days total where the battery was fully drained over night, and 3
days where the battery wasn't fully charged during the day. As we've changed
behaviour regarding electricity usage it's very difficult to say, but it's
absolutely clear that the addition of the battery makes a massive difference to
the benefit we get from the panels.
I want my own solar panels!
Go for it, if you can. One thing to note is that our installers told us there
is now huge interest - they're massively over subscribed with orders from their
expected plans for the year. So set your expectations accordingly in terms of
lead time.
Conclusion
These are the hard numbers from a single month, the real test will be to see
how we fare over a full year.
Comments? Reply to this tweet